Application
The App
class is the core of an ExpressoTS application, handling server creation and configuration.
It allows you to set up middleware and providers during the bootstrapping process and offers lifecycle hooks
to run code before, after, and during server shutdown.
App express
AppExpress
is the Express.js platform implementation specifically designed for ExpressoTS.
It integrates seamlessly with the Express.js ecosystem, providing a robust foundation for building
and configuring applications within ExpressoTS.
With full support for Express.js middleware, AppExpress
enables developers to efficiently manage application setup, execution, and shutdown processes,
all while leveraging the familiar Express.js environment.
Here is the App
class extending the AppExpress
adapter from the Opinionated template:
export class App extends AppExpress {
private config: AppContainer = this.configContainer([AppModule]);
protected globalConfiguration(): void | Promise<void> {
this.setGlobalRoutePrefix("/v1");
this.initEnvironment("development", {
env: {
development: ".env.development",
production: ".env.production",
},
});
}
protected configureServices(): void {
this.Provider.register(Env);
this.Middleware.addBodyParser();
this.Middleware.setErrorHandler({ showStackTrace: true });
}
protected async postServerInitialization(): Promise<void> {
if (this.isDevelopment()) {
this.Provider.get(Env).checkFile(".env.development");
}
}
protected serverShutdown(): void {}
}
-
this.Provider
: an object containing a register method that allows developers to register internal or external ExpressoTS-compatible providers. This method registers classes in the ExpressoTS container to be part of the dependency injection system, enabling the use of different scopes such as Transient, Scoped, and Singleton. -
this.Middleware
: offers a set of out-of-the-box middlewares that can be used to configure your application. See the complete list in the Middleware section
Be sure to explore other functionalities available in the class through this
, like setGlobalRoutePrefix(), setEngine(), and more.
Lifecycle hooks
An ExpressoTS application and its components follow a lifecycle managed by the framework. ExpressoTS offers lifecycle hooks that let developers track important events and run specific code within modules, providers, or controllers as these events happen.
The diagram below shows the sequence of key lifecycle events, from application startup to when the Node.js process exits.
async globalConfiguration(): Promise<void> { }
async configureServices(): Promise<void> { }
async postServerInitialization(): Promise<void> { }
async serverShutdown(): Promise<void> { }
Hooks execution order
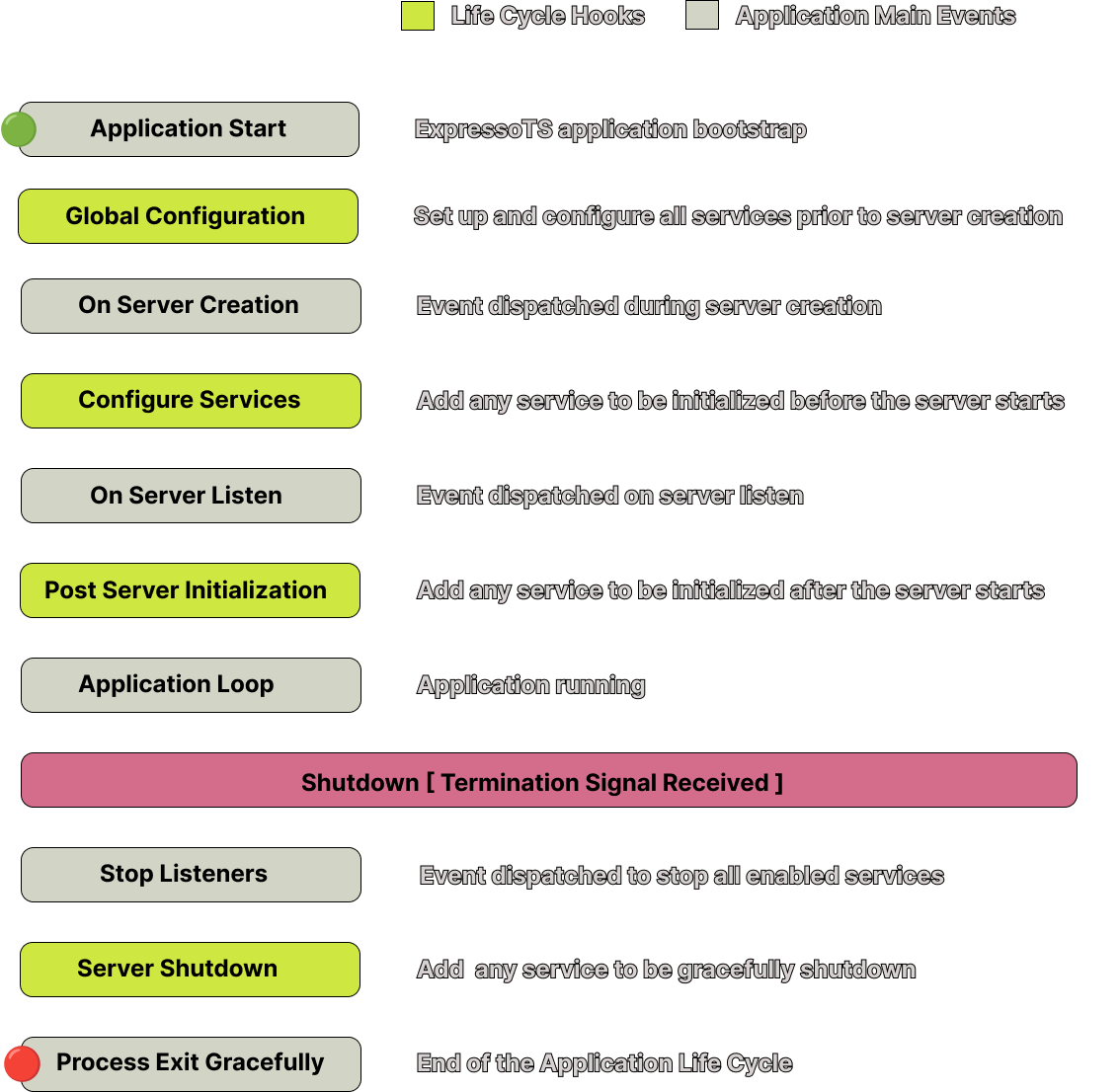
Method configContainer
The configContainer
method is used to configure the application container with the modules that will be used in the application.
private config: AppContainer = this.configContainer([AppModule]);
Middleware property
The Middleware
property is an instance of the MiddlewareManager
class, which provides a set of methods to add middleware to the application.
this.Middleware.addBodyParser();
Provider property
The Provider
property is an instance of the ProviderManager
class, which provides a set of methods to register and get providers in the application.
this.Provider.register(Env);
Method initEnvironment
The initEnvironment
method is used to set the environment variables for the application.
this.initEnvironment("development", {
env: {
development: ".env.development",
production: ".env.production",
},
});
Method isDevelopment
It is a helper method that returns a boolean value indicating whether the application is running in development mode. This method is useful for running specific code only in development environments.
this.isDevelopment(): boolean
Currently this method is being used in the postServerInitialization
method to check if the application is running in development mode.
If it is, the Env
provider is used to check all environment variables.
protected postServerInitialization(): void {
if (this.isDevelopment()) {
this.Provider.get(Env).checkFile(".env.development");
}
}
Method set global route prefix
You can set a global prefix for all routes in your application. This is useful when you want to version your APIs.
this.setGlobalRoutePrefix("/v1");
Method get http server
The getHttpServer
method is used to get the HTTP server instance.
this.getHttpServer(): http.Server
Method to set the engine
The setEngine
method is used to set the engine for the application.
this.setEngine(RenderEngine.Engine.EJS);
Support us ❤️
ExpressoTS is an MIT-licensed open source project. It's an independent project with ongoing development made possible thanks to your support. If you'd like to help, please read our support guide.